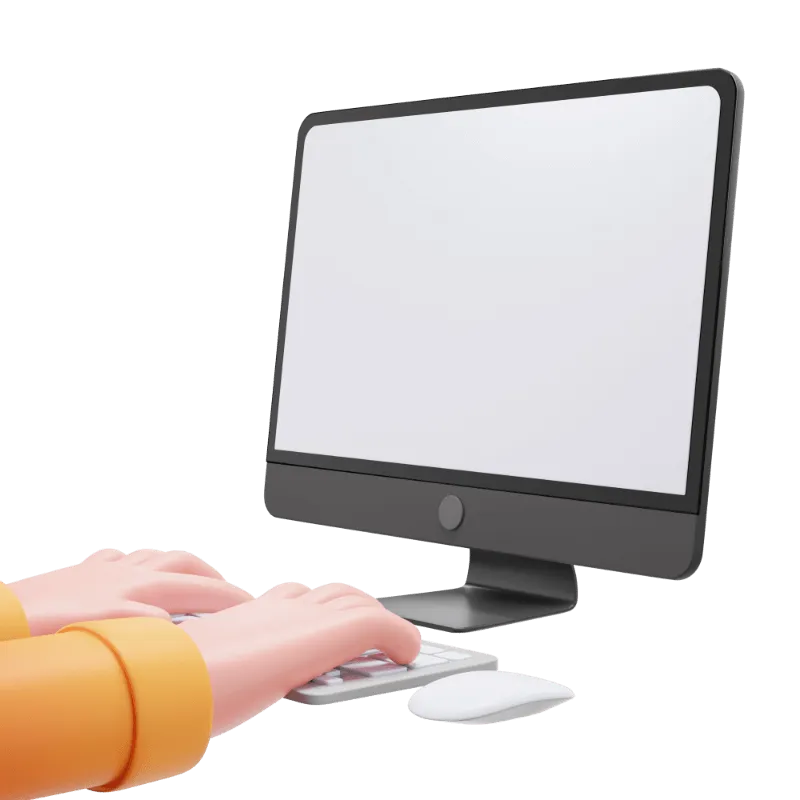
Existing member? Log in and continue learning
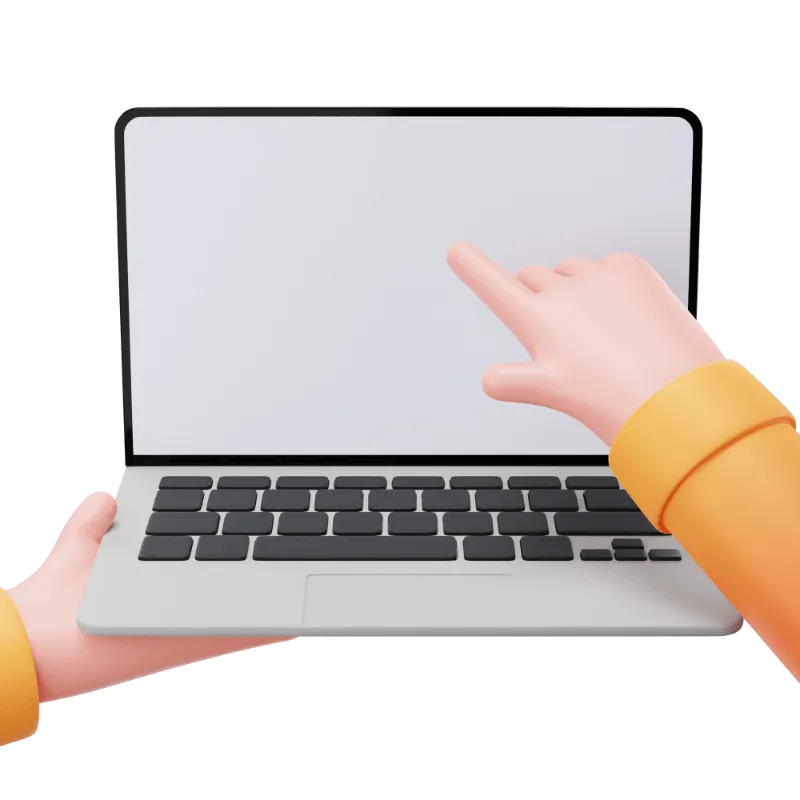
See if you like it, start the course for free!
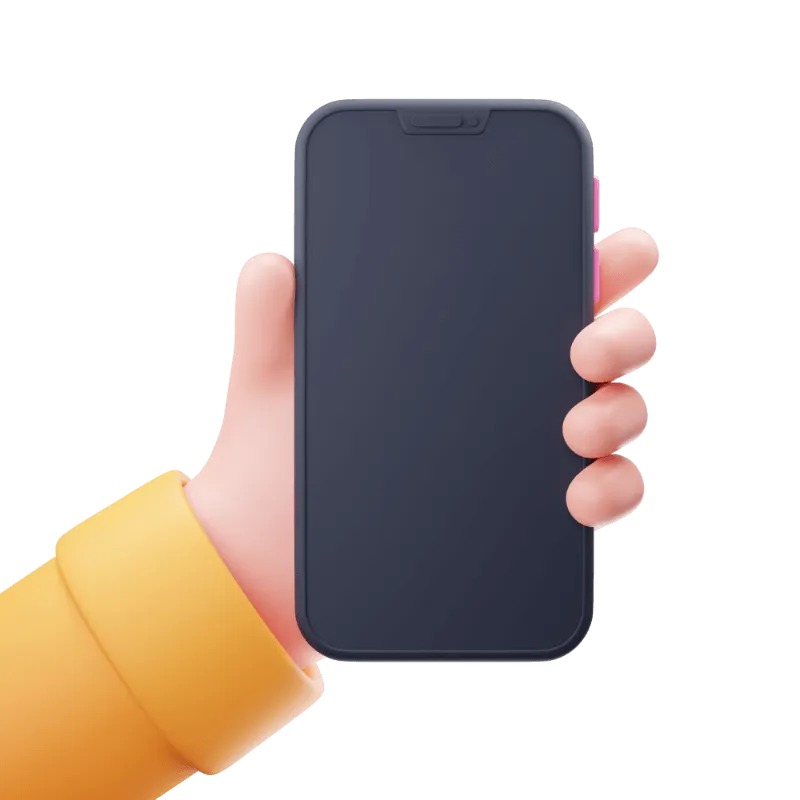
Unlock full course by purchasing a membership
Anatomy of an Angular Project
Anatomy of an Angular Project
In this lesson, we are going to discuss the general structure of the project we just created, but we will be leaving most of the discussion of how the project works as a whole to the Basic Angular Concepts and Syntax module.
The general idea in this lesson will just be to get our bearings a little, so that we don’t feel completely lost looking at a mess of files and folders in our newly generated project.
It will help for you to have your own project to poke around in. If you have not already generated a project on your machine, make sure to go back to the previous lesson and do that first.
Project Structure
Your newly generated Angular project should look something like this:
Directory.angular/
- …
Directory.vscode/
- …
Directorynode_modules/
- …
Directorysrc
Directoryapp
- app.component.css
- app.component.html
- app.component.spec.ts
- app.component.ts
- app.config.ts
- app.routes.ts
Directoryassets/
- …
- favicon.ico
- index.html
- main.ts
- styles.css
- .editorconfig
- .gitignore
- angular.json
- package-lock.json
- README.md
- tsconfig.app.json
- tsconfig.json
- tsconfig.spec.json
At first glance, there is an intimidating amount of stuff there — but you don’t need to know what everything does. We are going to talk through the files and folders that you will be using most frequently, and what they are responsible for. At this point, we just want to get a basic sense of the role of the different files and folders and we will discuss them in more depth later.
src
Perhaps the most important folder in your project is the src folder. This folder contains most of the files and folders that you will actually be working on to create your application. There are other files and folders outside of this, but you generally won’t need to touch those (much).
app
You will find the app folder inside of the src folder, and this folder holds a similar level of importance. Most of the code for your application will live inside of this app folder. As the application grows, we will add additional feature folders inside of the app folder — for example to hold features like the home page, detail page, checkout page, and so on.
We might have a checkout
feature at app/checkout
for example, or
a playlist
feature at app/playlist
. But, for now, we just have our root
app
files, which include:
app.component.ts
app.component.html
app.component.css
These define the default root component, which is sort of like the entry point
or container for your application. This one root component will contain your
entire application, and all the other features we create will be added inside of
it. It is similar in a sense to the way that the <body>
tag of an HTML page
contains the entire website — you could think of the <body>
tag as being like
a “root component” in this sense.
There are other app
files you might notice as well. You can ignore any spec
files you see — these are for the purpose of automated testing which is a more
advanced topic we will not be covering in this course.
However, we also have:
app.config.ts
app.routes.ts
The routes
define the routing information for the application — for example
this is where we might specify that if the user goes to the /playlist
URL then
we should load the playlist
component. For now, we just have an empty array
with no routes defined:
import { Routes } from '@angular/router';
export const routes: Routes = [];
The config
file as you might guess defines various configuration information
for the application. One such configuration is supplying the routes we create to
the router:
import { ApplicationConfig } from '@angular/core';import { provideRouter } from '@angular/router';
import { routes } from './app.routes';
export const appConfig: ApplicationConfig = { providers: [provideRouter(routes)],};
As we progress, you will find we supply other types of configurations here as
well. Although this is in a separate directory, it is also worth mentioning the
src/main.ts
file now too:
import { bootstrapApplication } from '@angular/platform-browser';import { appConfig } from './app/app.config';import { AppComponent } from './app/app.component';
bootstrapApplication(AppComponent, appConfig).catch((err) => console.error(err),);
This is what ties these two concepts together. The main.ts
file is what is
responsible for “bootstrapping”, or “creating”, or “launching” our application.
We supply it with our root component and our config.
Let’s come back to the root component now. We are going to talk more about this
in the Angular concepts module, but if you take a quick peek at
app.component.html
you will see a whole bunch of stuff. Most of this is just
some demo content with links to various resources. You can go ahead and delete
most of this, the only important thing in the file, and the only thing we will
keep when actually building the application, is this:
<router-outlet></router-outlet>
The <router-outlet>
is what controls what component is displayed to the user
based on the current route (i.e. the address in the URL bar).
Remember how I said the root component sort of “contains” the rest of the application?
This is the template for our root component, and from within our root component
we will want to display other components. If the user is on the /home
route,
then we probably want to display our Home component. The <router-outlet>
will handle detecting the current route, and then handle displaying the Home
component within this root component.
This is not exactly what it would look like in the DOM, but the general idea is that the template for our root component would now become something like this:
<router-outlet> <app-home-page></app-home-page></router-outlet>
If we then navigated to a different route, like /shop
then the
<router-outlet>
would handle removing the Home component and
displaying the Shop component instead:
<router-outlet> <app-shop-page></app-shop-page></router-outlet>
Again, we are going to discuss this in a lot more detail later but this is the basic idea. The router outlet handles displaying different components in the root component based on whatever the current route is.
assets
This folder is used to store any static assets for your application. This typically means images that you want to bundle with your application, but you can include any kind of static asset you like in here.
src/style.css
You might have noticed that our root component had its own .css
file. We can
apply styles to just specific components in their own style files, but we can
also place global styles in this style.css
file.
dist
This folder will only exist after you perform a build of your application. Go ahead and try it (make sure to run this inside of your project):
ng build
You won’t ever need to touch this folder, but it is important that you understand what it does. The code we write for our application is compiled into code that is understood by browsers. We are using TypeScript and Angular to create our application, but this code is not natively understood by the browser. When we build our application, it will generate standard JavaScript code that the browser can understand.
The dist folder contains the output of the build process for the application, and the code contained in this folder is the code that is actually run when a user uses the application. It is important not to make changes to the code in this folder, because it will be overwritten every time a new build is created.
If you were deploying your application to the web, it is the code in this folder
(specifically, the files inside of dist/my-app
) that would be uploaded to the
server hosting the application.
Review
As I mentioned, what we have discussed here is not all of the files/folders in your project, but these are the key ones that you will need to worry about. In the Angular concepts module, we will discuss in more depth how the application actually works.