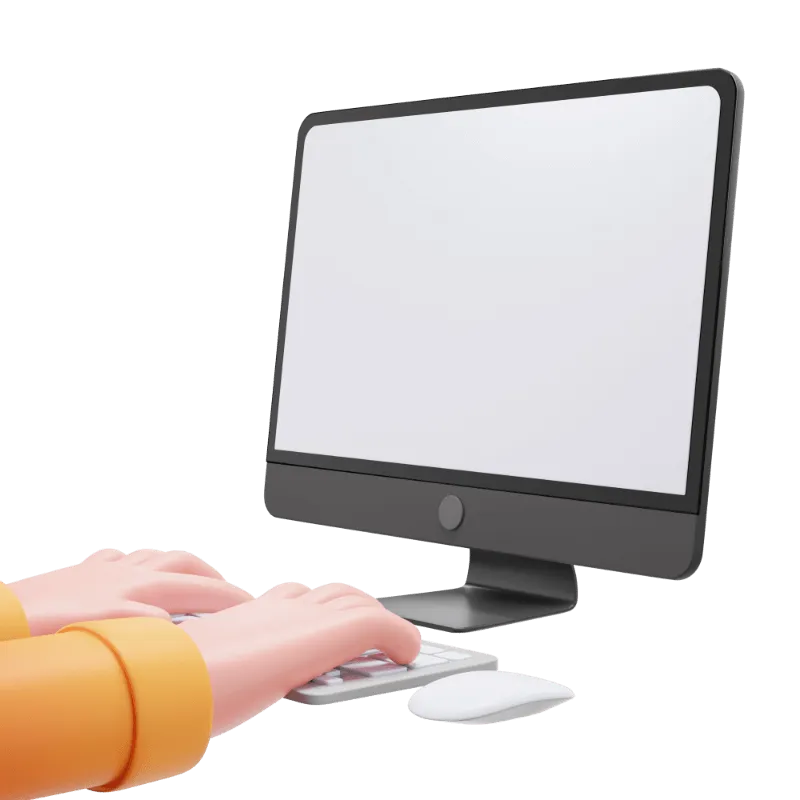
Existing member? Log in and continue learning
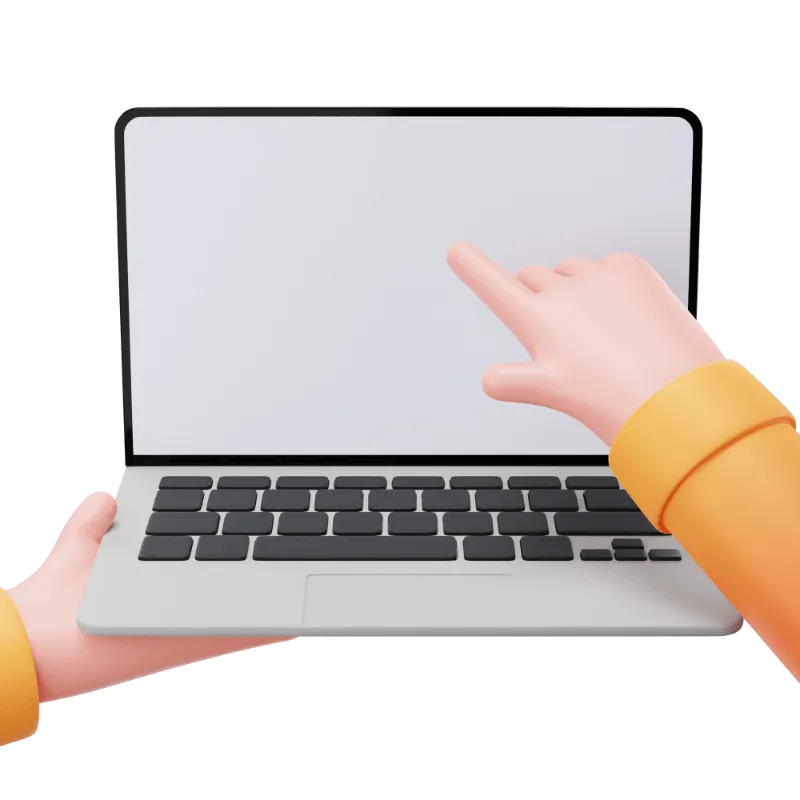
See if you like it, start the course for free!
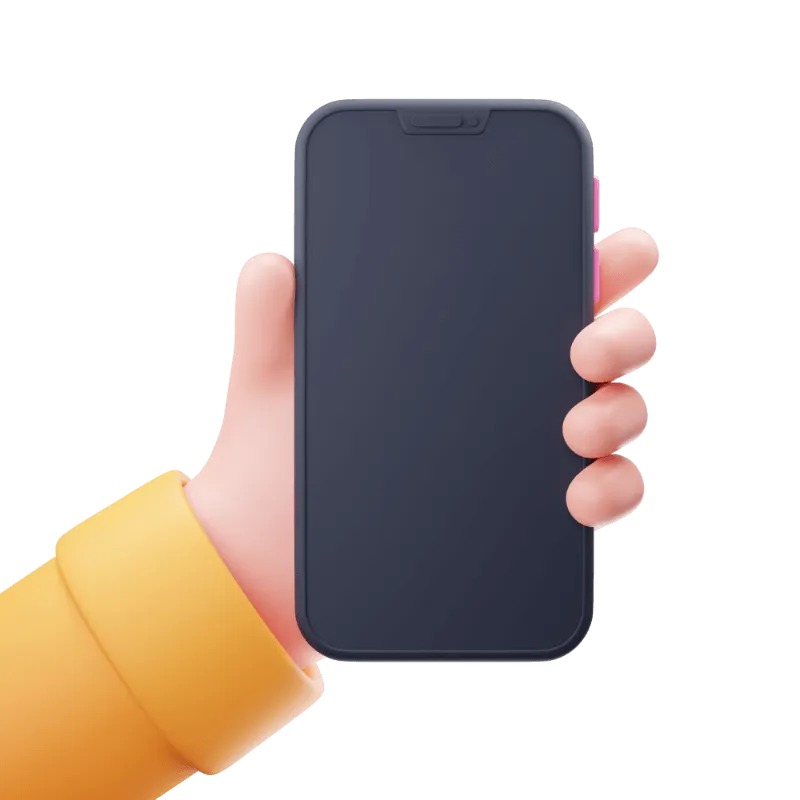
Unlock full course by purchasing a membership
Using the Angular CLI
Using the Angular CLI
The Angular CLI is a super powerful tool – we’ve already gone through how to use it to generate a new project and display your application in the browser, but there are a bunch more commands you should know about too, so let’s go through some of them. This is by no means an exhaustive list, but it will cover the most common and useful commands.
One thing to keep in mind for this course is that we are going to prefer to do things manually – e.g. rather than using the CLI to create a new component for us, we will create the files manually ourselves. I think this makes it seem a bit less magical and you will understand the “plumbing” of how things actually work.
Once you have a solid understanding of how things work, you can use the CLI to speed up your workflow. Personally, I still generally prefer to create things manually, but I am probably a bit of a weirdo in that regard.
Let’s get into it!
Help
You are not going to remember everything. You can always look at the documentation for information, but you can also run the help command:
ng --help
This will list the various commands you can run. You could then get further help
on a command by running help
for that command, e.g:
ng generate --help
This will show you all of the various generate
commands you can run.
Serving your Application
As you probably already know, you can view the Angular application that you are working on in the browser by running:
ng serve
Creating Applications
We have also already seen this command:
ng new
But there are also a bunch of flags/configuration we can pass to this command to generate applications with the specific configurations we want. For example, I already mentioned that I generally like to create my applications like this:
ng new my-app --defaults --style=scss --standalone --routing --inline-template --inline-style
If you run:
ng new --help
You can see a full list of the available flags and what they do.
Generate
When you are developing an application you will eventually want to add more
pages/components/services than the default ones that are created with the
application. To create more components you can manually create a new folder and
add the required files, or you can just run the ng generate
command to do it
automatically for you, with some handy boilerplate code in place.
The Angular CLI can create a lot of different things for us – here is just a few:
- component
- directive
- service
- resolver
- pipe
- config
- environments
- guard
To use the command you can just run something like (using the g
shorthand):
ng g component
and answer the prompt, or you can manually supply the name of your component/directive/service to the command like this (with optional configurations as well):
ng g component home
or:
ng g component home --style=none --inline-template --standalone
or:
ng g service shared/data-access/Settings
If you are running the manual command, make sure to supply the folder you want it to be generated in (e.g. as we have done for the service above) otherwise it will just be generated in the root folder of your application.
Environments
Angular projects used to come with environments by default, however, these are now generated manually with this generate command:
ng g environments
Run that now in a project and let’s look at what it does:
CREATE src/environments/environment.ts (31 bytes)CREATE src/environments/environment.development.ts (31 bytes)UPDATE angular.json (2823 bytes)
What this will allow us to do is define different configuration values depending
on whether our application is in development mode (e.g. we are just serving it),
or whether it is in production mode (e.g. it has been built with the ng build
command).
After running this generate command, Angular will handle automatically supplying
either the environment.ts
file or the environment.development.ts
file
depending on whether the application is in development or production. We aren’t
going to worry about this just now but, just to give you an idea, you might want
to use this so that you can use a development database/server in development,
but switch to the real one in production.
Creating a Build
As we just mentioned, when you want to create a production build of your application you can run this command:
ng build
Update
One of the great things about Angular is its commitment to long term support/stability and helping you manage updates.
Let’s say that you want to update your Angular application from v17
to v18
.
Instead of managing all the package updates yourself, you can run the update
command:
ng update @angular/cli @angular/core
This will update your project to the latest version of Angular. Keep in mind
that the Angular team recommends that you update one major version at
a time. Let’s say you haven’t touched your project in a while and now v20
is
out. In this circumstance, you should run the update
command for each major
version. For example, if you are currently on v17
you should run:
ng update @angular/cli@^18 @angular/core@^18
Make sure everything works as you expect, and then perform the next major upgrade:
ng update @angular/cli@^19 @angular/core@^19
…and so on. Generally, these updates are going to be easier if you keep your codebase reasonably up to date with whatever the latest Angular version is, but this is not always possible (Angular generally releases a new major version every 6 months).
Add
Another fantastic feature of the CLI is the add
command. When we want to add
some external package/library to our application this will involve installing
some npm
package. However, as well as that, we might also need to perform
other configurations in our application as well.
This command allows the authors of these packages/libraries to create
a schematic to do all/most of this automatically for us. For example, if we
wanted to add the @angular/fire
library to our project we could run:
ng add @angular/fire
…and it will automatically handle installing all of the required dependencies for us as well as guiding us through interactively configuring the application.